How do you perform an iterative inorder traversal of a binary tree?
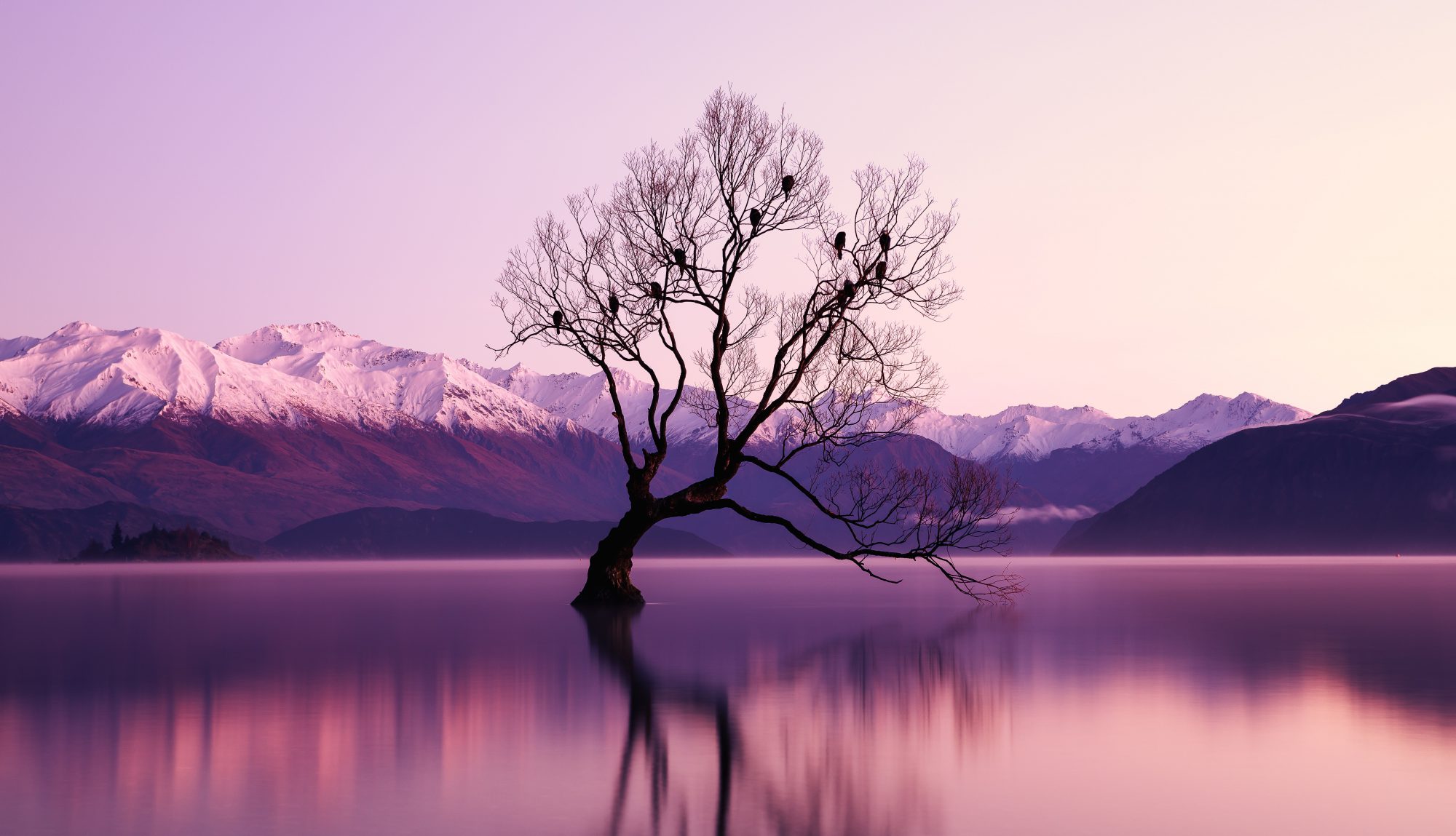
How do you perform an iterative inorder traversal of a binary tree?
How to perform an iterative inorder traversal of a binary tree
- Initialize an empty stack.
- Push the current node (starting from the root node) onto the stack.
- If the current node is NULL and the stack is not empty:
- If the current node is NULL and the stack is empty, then the algorithm has finished.
How do you traverse a binary search tree without recursion?
in-order:
- Create an empty stack S.
- Initialize current node as root.
- Push the current node to S and set current = current->left until current is NULL.
- If current is NULL and stack is not empty then. -> Pop the top item from stack.
- If current is NULL and stack is empty then we are done.
How do you traverse a given binary tree in preorder without recursion in Java?
Pre-order traversal in Java without recursion
- Create an empty stack.
- Push the root into Stack.
- Loop until Stack is empty.
- Pop the last node and print its value.
- Push right and left node if they are not null.
- Repeat from steps 4 to 6 again.
How do you print all nodes of a given binary tree using inorder traversal without recursion?
1) Create an empty stack S. 2) Initialize current node as root 3) Push the current node to S and set current = current->left until current is NULL 4) If current is NULL and stack is not empty then a) Pop the top item from stack. b) Print the popped item, set current = popped_item->right c) Go to step 3.
How do you iterate a tree iteratively?
What is recursive and non recursive tree traversing?
Recursive functions are simpler to implement since you only have to care about a node, they use the stack to store the state for each call. Non-recursive functions have a lot less stack usage but require you to store a list of all nodes for each level and can be far more complex than recursive functions.
How do you iterate all nodes in a binary tree?
Here’s how it can be defined:
- First rule: The first node in the tree is the leftmost node in the tree.
- Next rule: The successor of a node is: Next-R rule: If it has a right subtree, the leftmost node in the right subtree. Next-U rule: Otherwise, traverse up the tree.
How to iteratively traverse a binary tree?
First,traverse the left subtree
How to build binary tree from post order?
Construct the root node of BST,which would be the last key in the postorder sequence.
How do I iterate over binary trees?
If you make a right turn (i.e. this node was a left child),then that parent node is the successor
What is the Order of binary tree?
The order of binary tree is ‘2’. Binary tree does not allow duplicate values. While constructing a binary, if an element is less than the value of its parent node, it is placed on the left side of it otherwise right side. A binary tree is shown for the element 40, 56, 35, 48, 22, 65, 28.