How do I unzip a gzip file in Python?
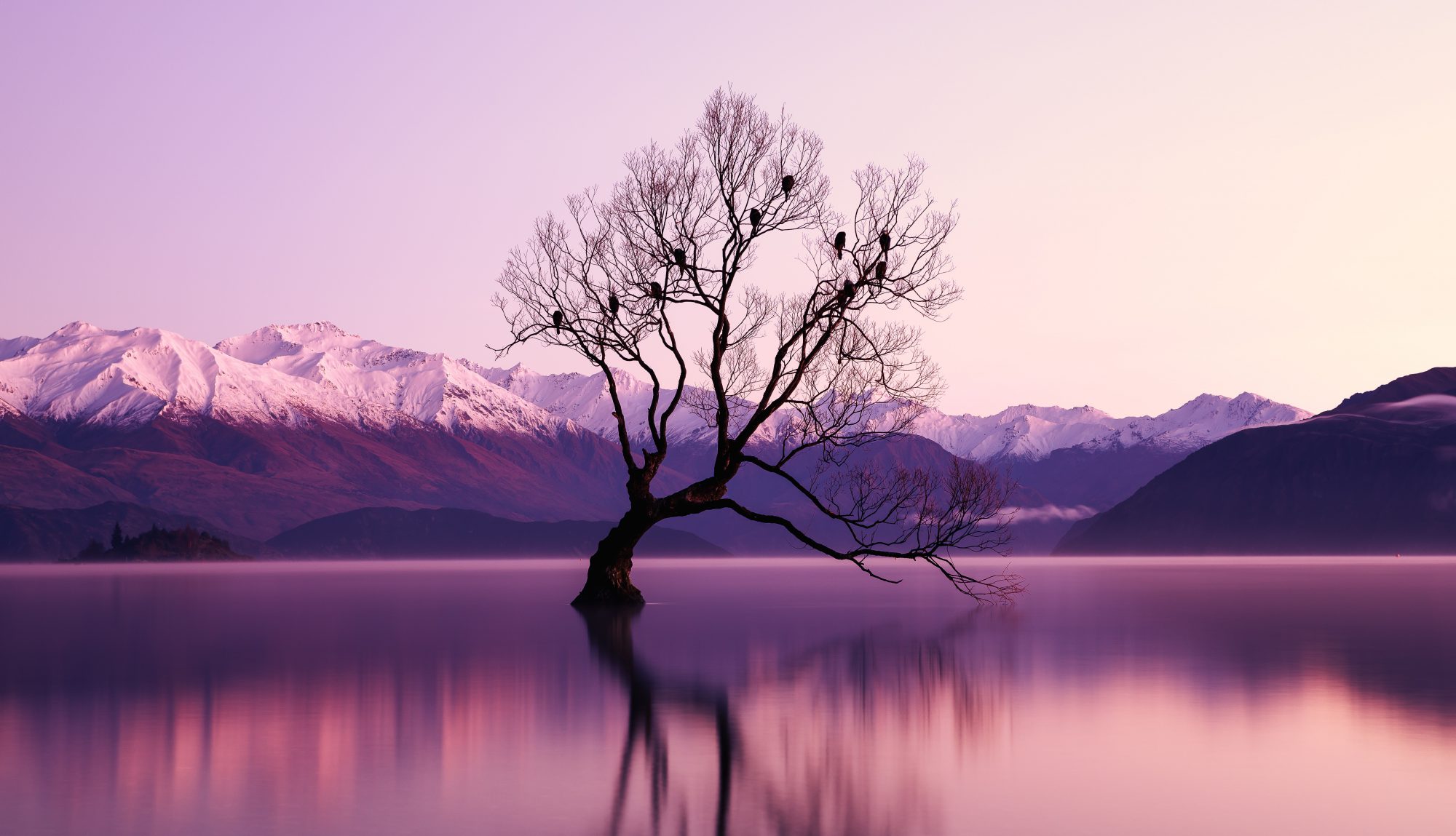
How do I unzip a gzip file in Python?
Easiest way to achieve compression and decompression is by using above mentioned functions.
- open() This function opens a gzip-compressed file in binary or text mode and returns a file like object, which may be physical file, a string or byte object.
- compress()
- decompress()
How do I extract a gzip file?
How to open GZIP files
- Save the .
- Launch WinZip from your start menu or Desktop shortcut.
- Select all the files and folders inside the compressed file.
- Click 1-click Unzip and choose Unzip to PC or Cloud in the WinZip toolbar under the Unzip/Share tab.
What does gzip do in Python?
The gzip module provides the GzipFile class, as well as the open() , compress() and decompress() convenience functions. The GzipFile class reads and writes gzip-format files, automatically compressing or decompressing the data so that it looks like an ordinary file object.
How do I unzip a CSV gz file in Python?
“unzip gz file python” Code Answer
- import gzip.
- import shutil.
- with gzip. open(‘file.txt.gz’, ‘rb’) as f_in:
- with open(‘file.txt’, ‘wb’) as f_out:
- shutil. copyfileobj(f_in, f_out)
How do I read a zip file in Python?
We create a ZipFile object in READ mode and name it as zip. printdir() method prints a table of contents for the archive. extractall() method will extract all the contents of the zip file to the current working directory. You can also call extract() method to extract any file by specifying its path in the zip file.
How do I compress and uncompress a file in Python?
To create your own compressed ZIP files, you must open the ZipFile object in write mode by passing ‘w’ as the second argument. When you pass a path to the write() method of a ZipFile object, Python will compress the file at that path and add it into the ZIP file.
How do I open a .gz file without extracting it?
Just use zcat to see content without extraction. From the manual: zcat is identical to gunzip -c . (On some systems, zcat may be installed as gzcat to preserve the original link to compress .)
What is a gz file and how do I open it?
A GZ file is an archive file compressed by the standard GNU zip (gzip) compression algorithm. It typically contains a single compressed file but may also store multiple compressed files. gzip is primarily used on Unix operating systems for file compression.
How do I unzip a file in Python?
To unzip a file in Python, use the ZipFile. extractall() method. The extractall() method takes a path, members, pwd as an argument and extracts all the contents.
How do I open a gzip file in CSV?
Unzip downloaded . gzip, csv. gz, or . zip file
- Navigate to your download folder.
- Double-click the file.
- Your operating system will automatically unzip to correct format.
How do you unzip a list in Python?
How to Unzip List of Tuples in Python
- Python zip() method returns the zip object, which is an iterator of tuples.
- To unzip a list of tuples means it returns the new list of tuples where each tuple consists of the identical items from each original tuple.
How do I view the contents of a gzip file?
How to read Gzip compressed files in Linux command line
- zcat for cat to view compressed file.
- zgrep for grep to search inside the compressed file.
- zless for less, zmore for more, to view the file in pages.
- zdiff for diff to see the difference between two compressed files.
How do I zip a file in Python 3?
Summary
- To zip entire directory use command “shutil.make_archive(“name”,”zip”, root_dir)
- To select the files to zip use command “ZipFile.write(filename)”
How do I extract multiple ZIP files in Python?
Python: How to unzip a file | Extract Single, multiple or all files from a ZIP archive
- ZipFile. extractall(path=None, members=None, pwd=None) ZipFile.extractall(path=None, members=None, pwd=None)
- from zipfile import ZipFile. from zipfile import ZipFile.
- ZipFile. extract(member, path=None, pwd=None)
How do I read a compressed CSV file in Python?
“how to read zip csv file in python” Code Answer
- import pandas as pd.
- import zipfile.
-
- zf = zipfile. ZipFile(‘C:/Users/Desktop/THEZIPFILE.zip’)
- # if you want to see all files inside zip folder.
- zf. namelist()
- # now read your csv file.
- df = pd. read_csv(zf. open(‘intfile.csv’))