How do I compress a gzip file in Java?
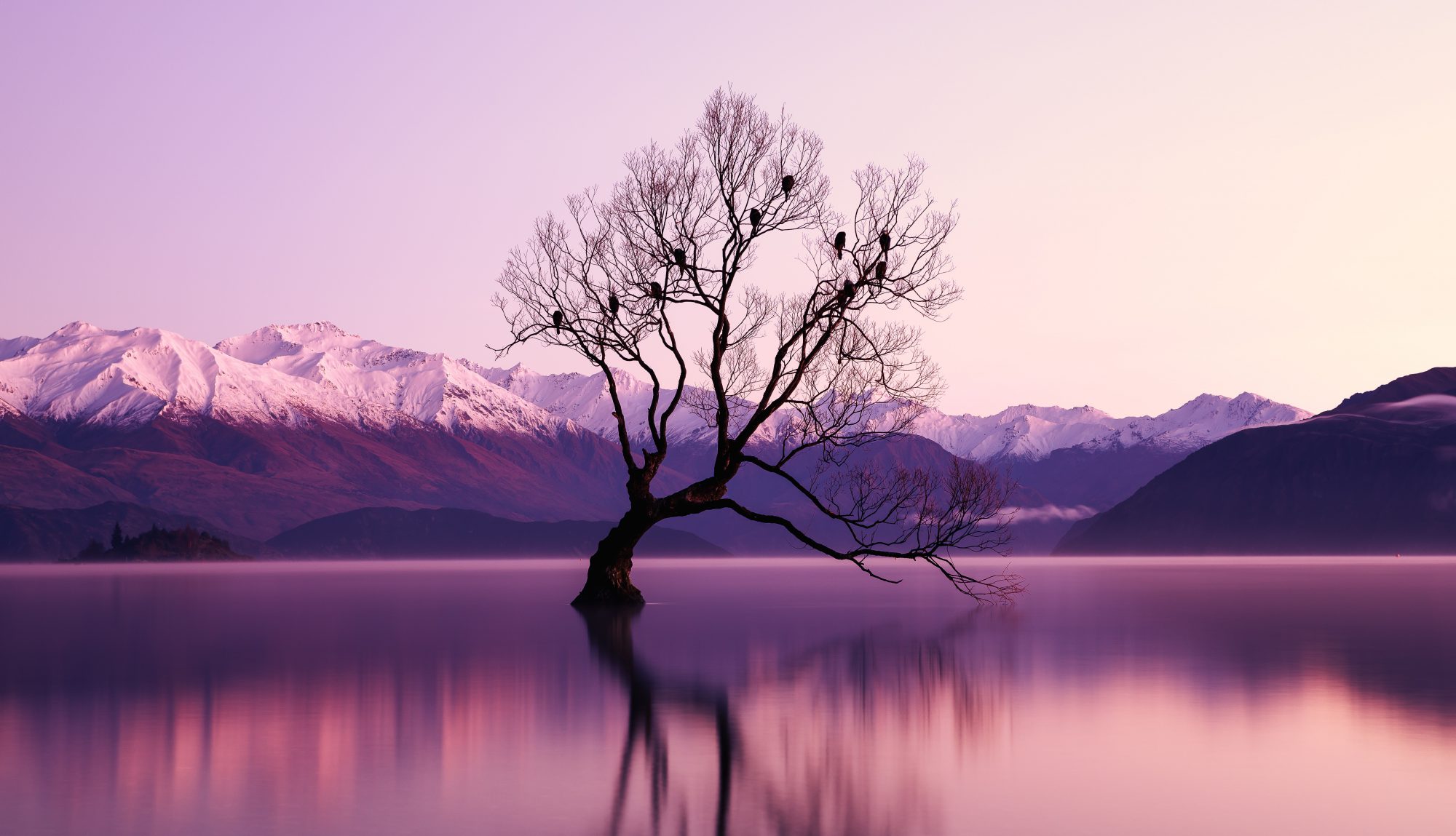
How do I compress a gzip file in Java?
Compress a File in GZIP format in Java
- Create a FileOutputStream to the destination file, that is the file path to the output compressed file.
- Create a GZIPOutputStream to the above FileOutputStream .
- Create a FileInputStream to the file you want to compress.
How do you compress and decompress the data of a file in Java?
Steps to compress a file(file 1)
- Take an input file ‘file 1’ to FileInputStream for reading data.
- Take the output file ‘file 2’ and assign it to FileOutputStream .
- Assign FileOutputStream to DeflaterOutputStream for Compressing the data.
- Now, read data from FileInputStream and write it into DeflaterOutputStream.
How do you compress an object in Java?
Java object compression is done using the GZIPOutputStream class (this class implements a stream filter for writing compressed data in the GZIP file format) and passes it to the ObjectOutputStream class (this class extends OutputStream and implements ObjectOutput, ObjectStreamConstants) to write the object into an …
How do I compress a java project?
3 Answers
- File > Export.
- Select the General > Archive File export wizard.
- Select the project(s) to be exported.
- Choose the archive file type (ZIP or TAR), and other options.
- Enter the archive file name.
- Click Finish.
What is GZIPInputStream in Java?
GZIPInputStream(InputStream in) Creates a new input stream with a default buffer size. GZIPInputStream(InputStream in, int size) Creates a new input stream with the specified buffer size.
What is Compressedoops?
Compressed oops represent managed pointers (in many but not all places in the JVM) as 32-bit values which must be scaled by a factor of 8 and added to a 64-bit base address to find the object they refer to. This allows applications to address up to four billion objects (not bytes), or a heap size of up to about 32Gb.
Is gz and gzip same?
A GZ file is an archive file compressed by the standard GNU zip (gzip) compression algorithm. It typically contains a single compressed file but may also store multiple compressed files. gzip is primarily used on Unix operating systems for file compression.
How do I compress a jar file?
A jar (and war and ear) are . zip archives that have a specific directory and file structure under them. As they are zipped, the only way to further compress the contents is to remove things from them.
How do I zip a project file?
Press and hold (or right-click) the file or folder, select (or point to) Send to, and then select Compressed (zipped) folder. A new zipped folder with the same name is created in the same location.
How do I compress a large string in Java?
Following are the changes which need to be done in your code. byte[] compressed = compress(string); //In the main method public static byte[] compress(String str) throws Exception { … return obj. toByteArray(); } public static String decompress(byte[] bytes) throws Exception { …
How do you DEFLATE a string in Java?
deflate(byte[] b) method compresses the input data and fills specified buffer with compressed data. Returns actual number of bytes of compressed data. A return value of 0 indicates that needsInput should be called in order to determine if more input data is required.
Who developed ZIP Close method?
Forty-two years later, in 1893, Whitcomb Judson, who invented a pneumatic street railway, patented a “Clasp Locker”. The device served as a (more complicated) hook-and-eye shoe fastener. With the support of businessman Colonel Lewis Walker, Judson launched the Universal Fastener Company to manufacture the new device.
What is a gzip file in Java?
Welcome to Java GZIP example. GZIP is one of the favorite tool to compress file in Unix systems. We can compress a single file in GZIP format but we can’t compress and archive a directory using GZIP like ZIP files.
How do I create a gzip input stream in Java?
Creating a GZIPInputStream To use the Java GZIPInputStream you must first create a GZIPInputStream instance. Here is an example of creating a GZIPInputStream instance: InputStream fileInputStream = new FileInputStream (“myfile.zip”); GZIPInputStream gzipInputStream = new GZIPInputStream (fileInputStream);
How to compress and decompress gzip file?
Compress GZIP file: We use the GZIPOutputStream to write the compressed GZIP file. Decompress GZIP file: We use the GZIPInputStream to read the compressed GZIP file. You can also use org.apache.commons:commons-compress dependency to compress and decompress to and from GZIP formats.
How do I use the Java gzipinputstream class to decompress files?
The Java GZIPInputStream class ( java.util.zip.GZIPInputStream) can be used to decompress files that are compressed with the GZIP compression algorithm, for instance via the GZIPOutputStream class. To use the Java GZIPInputStream you must first create a GZIPInputStream instance. Here is an example of creating a GZIPInputStream instance: