How to parse string to ZonedDateTime in java?
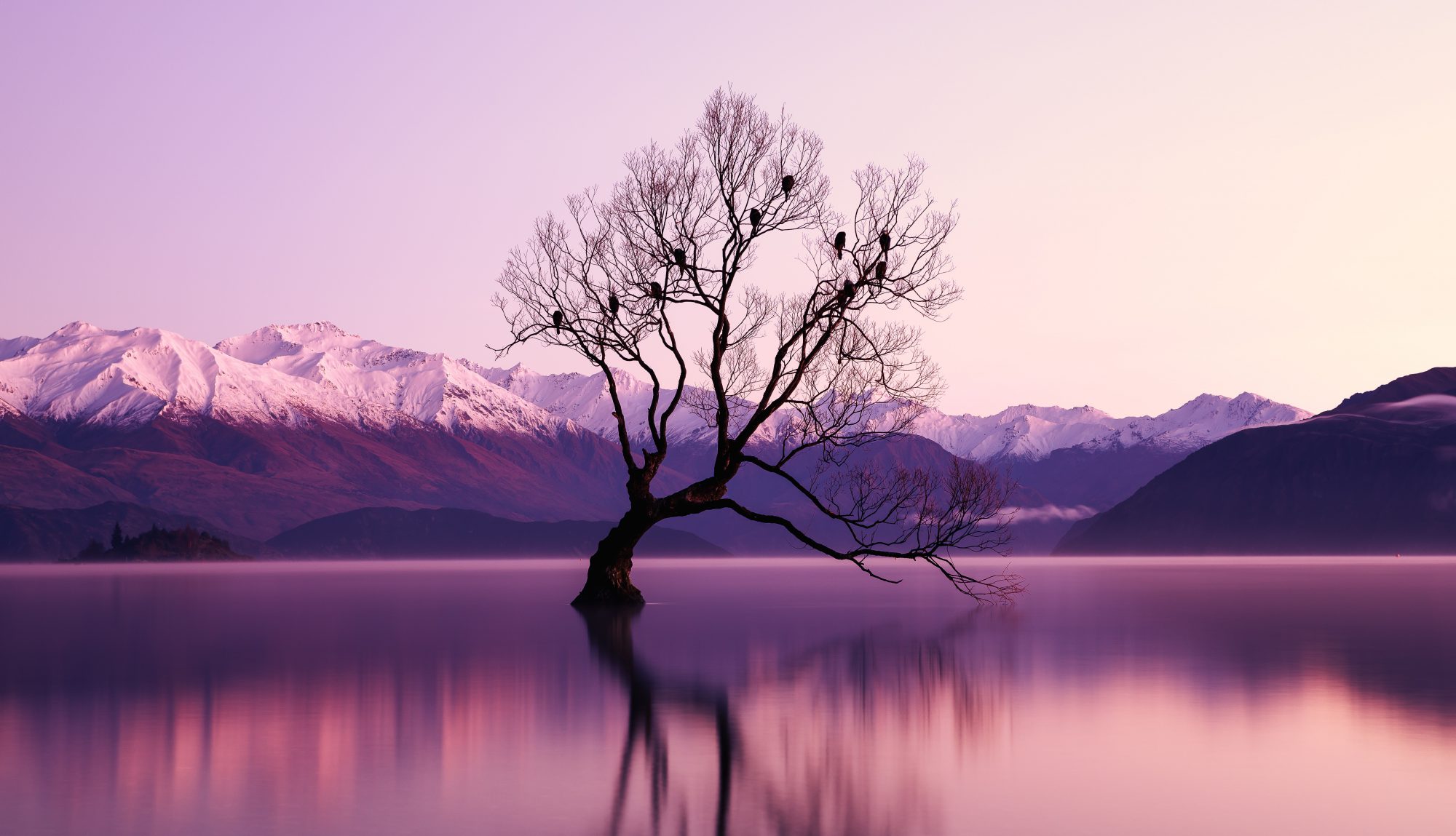
How to parse string to ZonedDateTime in java?
Converting a String to ZonedDateTime Converting a String to a ZonedDateTime object is as simple as: ZonedDateTime zonedDateTime = ZonedDateTime. parse(“2018-09-16T08:00:00+00:00[Europe/London]”);
What is ZonedDateTime?
ZonedDateTime is an immutable representation of a date-time with a time-zone. This class stores all date and time fields, to a precision of nanoseconds, and a time-zone, with a zone offset used to handle ambiguous local date-times. For example, the value “2nd October 2007 at 13:45.30.
How does ZonedDateTime work?
Methods of Java ZonedDateTime It is used to return a copy of this date-time with a different time-zone, retaining the instant. It is used to obtain the current date-time from the system clock in the default time-zone. It is used to obtain an instance of ZonedDateTime from a local date and time.
How do I change LocalDateTime format?
How to Format LocalDateTime in Java 8
- DateTimeFormatter. To format LocalDateTime , use DateTimeFormatter available in the new time API.
- String to LocaDateTime. To convert String to LocaDateTime , use the static parse() method available with LocalDateTime class.
- Parsing String with ISO-8601 Format.
What is a LocalDateTime?
LocalDateTime ) represents a local date and time without any time zone information. You could view the LocalDateTime as a combination of the LocalDate and LocalTime classes of the Java 8 date time API.
How do I parse LocalDateTime to ZonedDateTime?
Convert LocalDateTime to ZonedDateTime The LocalDateTime has no time zone; to convert the LocalDateTime to ZonedDateTime , we can use . atZone(ZoneId. systemDefault()) to create a ZonedDateTime containing the system default time zone and convert it to another time zone using a predefined zone id or offset.
How do I convert OffsetDateTime to instant?
Simply do this:
- OffsetDateTime odt = instant. atOffset( myZoneOffset ) ;
- ZonedDateTime zdt = instant. atZone( myZoneId ) ;
- OffsetDateTime odt = zdt.toOffsetDateTime() ;
How do I bypass LocalDateTime?
Java LocalDateTime Example: now()
- import java.time.LocalDateTime;
- import java.time.format.DateTimeFormatter;
- public class LocalDateTimeExample2 {
- public static void main(String[] args) {
- LocalDateTime datetime1 = LocalDateTime.now();
- DateTimeFormatter format = DateTimeFormatter.ofPattern(“dd-MM-yyyy HH:mm:ss”);
What is the difference between OffsetDateTime and ZonedDateTime?
Instant is the simplest, simply representing the instant. OffsetDateTime adds to the instant the offset from UTC/Greenwich, which allows the local date-time to be obtained. ZonedDateTime adds full time-zone rules. It is intended that ZonedDateTime or Instant is used to model data in simpler applications.
What is OffsetDateTime in Java?
Java OffsetDateTime class is an immutable representation of a date-time with an offset. It inherits Object class and implements the Comparable interface. OffsetDateTime class is used to store the date and time fields, to a precision of nanoseconds.